Note
You are not reading the most up to date version of Gammapy documentation.
Access the latest stable version v1.3 or the list of Gammapy releases.
Note
A new set of map and cube classes is being developed in gammapy.maps
and long-term will replace the existing gammapy.image.SkyImage
and
gammapy.cube.SkyCube
classes. Please consider trying out gammapy.maps
and changing your scripts to use those new classes. See Data Structures for Images and Cubes (gammapy.maps).
Image processing and analysis tools (gammapy.image
)¶
Introduction¶
gammapy.image
contains data classes and methods for image based analysis
of gamma-ray data.
Getting Started¶
The central data structure in gammapy.image
is the SkyImage
class, which combines the raw data with WCS information, FITS I/O functionality
and many other methods, that allow easy handling, processing and plotting of
image based data. Here is a first example:
from gammapy.image import SkyImage
filename = '$GAMMAPY_EXTRA/datasets/fermi_2fhl/fermi_2fhl_vela.fits.gz'
image = SkyImage.read(filename, hdu=2)
image.show()
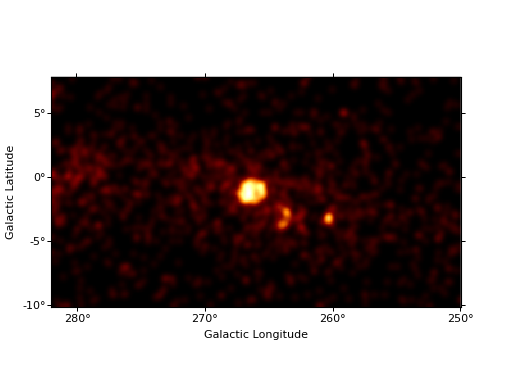
This loads a prepared Fermi 2FHL FITS image of the Vela region, creates a
SkyImage
and shows it on the the screen by calling SkyImage.show()
.
To explore further the SkyImage class try tab completion on the image
object
in an interactive python environment or see the Sky image page.
Using gammapy.image
¶
Many of the Gammapy tutorial notebooks show examples using gammapy.image
:
Documentation pages with more detailed information:
Reference/API¶
gammapy.image Package¶
Sky images (2-dimensional: lon, lat).
Functions¶
asmooth_scales (n_scales[, factor, kernel]) |
Create list of Gaussian widths. |
block_reduce_hdu (input_hdu, block_size, func) |
Provides block reduce functionality for image HDUs. |
colormap_hess ([transition, width]) |
Colormap often used in H.E.S.S. |
colormap_milagro ([transition, width, huestart]) |
Colormap often used in Milagro collaboration publications. |
fits_to_png (infile, outfile, draw[, dpi]) |
Plot FITS image in PNG format. |
fitsfigure_add_psf_inset (ff, psf, box[, …]) |
Add PSF inset to FITSFigure instance. |
grayify_colormap (cmap[, mode]) |
Return a grayscale version a the colormap. |
illustrate_colormap (cmap, **kwargs) |
Illustrate color distribution and perceived luminance of a colormap. |
image_groupby (images, labels) |
Group pixel by labels. |
lon_lat_circle_mask (lons, lats, center_lon, …) |
Produces a circular boolean mask array. |
lon_lat_rectangle_mask (lons, lats[, …]) |
Produces a rectangular boolean mask array based on lat and lon limits. |
make_header ([nxpix, nypix, binsz, xref, …]) |
Generate a FITS header from scratch. |
measure_containment (image, position, radius) |
Measure containment in a given circle around the source position. |
measure_containment_fraction (image, radius, …) |
Measure containment fraction. |
measure_containment_radius (image, position) |
Measure containment radius of a source. |
measure_curve_of_growth (image, position[, …]) |
Measure the curve of growth for a given source position. |
measure_image_moments (image) |
Compute 0th, 1st and 2nd moments of an image. |
measure_labeled_regions (data, labels[, tag, …]) |
Measure source properties in image. |
process_image_pixels (images, kernel, out, …) |
Process images for a given kernel and per-pixel function. |
radial_profile (image, center, radius) |
Image radial profile. |
radial_profile_label_image (image, center, radius) |
Image radial profile label image. |
Classes¶
ASmooth ([kernel, method, threshold, scales]) |
Adaptively smooth counts image. |
BasicImageEstimator |
BasicImageEstimator base class. |
CatalogImageEstimator (reference, emin, emax) |
Compute model image for given energy band from a catalog. |
FermiLATBasicImageEstimator (reference, emin, …) |
Estimate basic sky images for Fermi-LAT data. |
GalacticPlaneSurveyPanelPlot ([npanels, …]) |
Plot Galactic plane survey images in multiple panels. |
IACTBasicImageEstimator (reference, emin, emax) |
Estimate the basic sky images for a set of IACT observations. |
ImageProfile (table) |
Image profile class. |
ImageProfileEstimator ([x_edges, method, axis]) |
Estimate profile from image. |
SkyImage ([name, data, wcs, unit, meta]) |
Sky image. |
SkyImageList ([images, meta]) |
List of SkyImage objects. |
gammapy.image.models Package¶
Morphology models.
Functions¶
gaussian_sum_moments (F, sigma, x, y, cov_matrix) |
Compute image moments with uncertainties for sum of Gaussians. |
Classes¶
Delta2D (amplitude, x_0, y_0, **constraints) |
Two dimensional delta function . |
Gauss2DPDF ([sigma]) |
2D symmetric Gaussian PDF. |
ModelThetaCalculator (source, psf, fov, binsz) |
Compute containment radius for given radially symmetric source and psf as well as desired containment fraction. |
MultiGauss2D (sigmas[, norms]) |
Sum of multiple 2D Gaussians. |
Shell2D (amplitude, x_0, y_0, r_in[, width, …]) |
Projected homogeneous radiating shell model. |
Sphere2D (amplitude, x_0, y_0, r_0[, normed]) |
Projected homogeneous radiating sphere model. |
Template2D (image[, amplitude, frame]) |
Two dimensional table model. |
ThetaCalculator (dist, theta_max, n_bins[, …]) |
Provides methods containment_fraction(theta) and containment_radius(containment_fraction) given some 1D distribution (not necessarily normalized). |
ThetaCalculator2D (dist, x, y) |
Methods to compute theta and containment for a given 2D probability distribution image. |
ThetaCalculatorScipy (dist, theta_max[, …]) |
Same functionality as NumericalThetaCalculator, but uses scipy.integrate.quad and scipy.optimize.fsolve instead. |
Variables¶
morph_types |
Spatial model registry (OrderedDict ). |