Note
Click here to download the full example code or to run this example in your browser via Binder
Piecewise norm spectral model#
This model parametrises a piecewise spectral correction with a free norm parameter at each fixed energy node.
Example plot#
Here is an example plot of the model:
from astropy import units as u
import matplotlib.pyplot as plt
from gammapy.modeling.models import (
Models,
PiecewiseNormSpectralModel,
PowerLawSpectralModel,
SkyModel,
)
energy_bounds = [0.1, 100] * u.TeV
model = PiecewiseNormSpectralModel(
energy=[0.1, 1, 3, 10, 30, 100] * u.TeV,
norms=[1, 3, 8, 10, 8, 2],
)
model.plot(energy_bounds, yunits=u.Unit(""))
plt.grid(which="both")
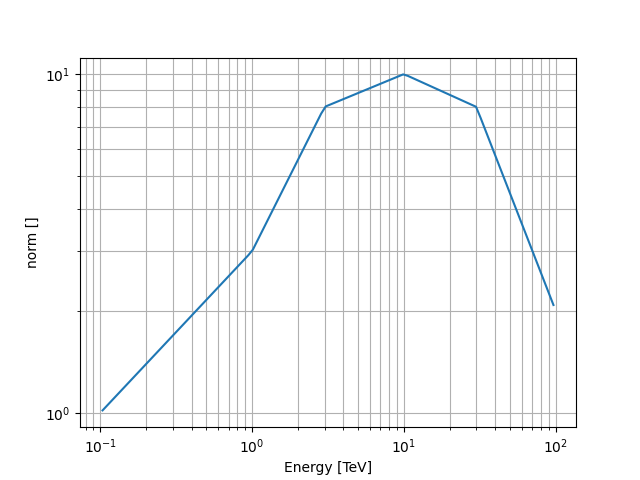
YAML representation#
Here is an example YAML file using the model:
model = model * PowerLawSpectralModel()
model = SkyModel(spectral_model=model, name="piecewise-norm-model")
models = Models([model])
print(models.to_yaml())
components:
- name: piecewise-norm-model
type: SkyModel
spectral:
type: CompoundSpectralModel
model1:
type: PiecewiseNormSpectralModel
parameters:
- name: norm_0
value: 1.0
- name: norm_1
value: 3.0
- name: norm_2
value: 8.0
- name: norm_3
value: 10.0
- name: norm_4
value: 8.0
- name: norm_5
value: 2.0
energy:
data:
- 0.1
- 1.0
- 3.0
- 10.0
- 30.0
- 100.0
unit: TeV
model2:
type: PowerLawSpectralModel
parameters:
- name: index
value: 2.0
- name: amplitude
value: 1.0e-12
unit: cm-2 s-1 TeV-1
- name: reference
value: 1.0
unit: TeV
operator: mul