Note
Click here to download the full example code or to run this example in your browser via Binder
Template spectral model#
This model is defined by custom tabular values.
The units returned will be the units of the values array provided at initialization. The model will return values interpolated in log-space, returning 0 for energies outside of the limits of the provided energy array.
The class implementation follows closely what has been done in
naima.models.TemplateSpectralModel
Example plot#
Here is an example plot of the model:
import numpy as np
from astropy import units as u
import matplotlib.pyplot as plt
from gammapy.modeling.models import (
Models,
PowerLawNormSpectralModel,
SkyModel,
TemplateSpectralModel,
)
energy_bounds = [0.1, 1] * u.TeV
energy = np.array([1e6, 3e6, 1e7, 3e7]) * u.MeV
values = np.array([4.4e-38, 2.0e-38, 8.8e-39, 3.9e-39]) * u.Unit("MeV-1 s-1 cm-2")
template = TemplateSpectralModel(energy=energy, values=values)
template.plot(energy_bounds)
plt.grid(which="both")
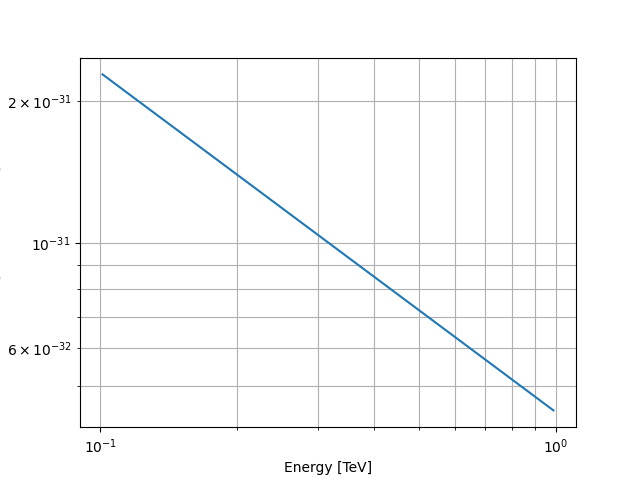
Spectral correction
Corrections to templates can be applied by multiplication with a normalized spectral model,
for example gammapy.modeling.models.PowerLawNormSpectralModel
.
This operation create a new gammapy.modeling.models.CompoundSpectralModel
new_model = template * PowerLawNormSpectralModel(norm=2, tilt=0)
print(new_model)
CompoundSpectralModel
Component 1 : TemplateSpectralModel
type name value unit error min max frozen is_norm link
-------- ---- ---------- ---- --------- --- --- ------ ------- ----
spectral norm 1.0000e+00 0.000e+00 nan nan True True
Component 2 : PowerLawNormSpectralModel
type name value unit error min max frozen is_norm link
-------- --------- ---------- ---- --------- --- --- ------ ------- ----
spectral norm 2.0000e+00 0.000e+00 nan nan False True
spectral tilt 0.0000e+00 0.000e+00 nan nan True False
spectral reference 1.0000e+00 TeV 0.000e+00 nan nan True False
Operator : mul
YAML representation#
Here is an example YAML file using the model:
components:
- name: template-model
type: SkyModel
spectral:
type: TemplateSpectralModel
parameters:
- name: norm
value: 1.0
energy:
data:
- 1000000.0
- 3000000.0
- 10000000.0
- 30000000.0
unit: MeV
values:
data:
- 4.4e-38
- 2.0e-38
- 8.8e-39
- 3.9e-39
unit: 1 / (cm2 MeV s)