Note
Click here to download the full example code
Template Spectral Model¶
This model is defined by custom tabular values.
The units returned will be the units of the values array provided at initialization. The model will return values interpolated in log-space, returning 0 for energies outside of the limits of the provided energy array.
The class implementation follows closely what has been done in
naima.models.TemplateSpectralModel
Example plot¶
Here is an example plot of the model:
import numpy as np
from astropy import units as u
import matplotlib.pyplot as plt
Spectral correction
Corrections to templates can be applied by multiplication with a normalized spectral model,
for example gammapy.modeling.models.PowerLawNormSpectralModel
.
This operation create a new gammapy.modeling.models.CompoundSpectralModel
from gammapy.modeling.models import (
Models,
PowerLawNormSpectralModel,
SkyModel,
TemplateSpectralModel,
)
energy_range = [0.1, 1] * u.TeV
energy = np.array([1e6, 3e6, 1e7, 3e7]) * u.MeV
values = np.array([4.4e-38, 2.0e-38, 8.8e-39, 3.9e-39]) * u.Unit("MeV-1 s-1 cm-2")
template = TemplateSpectralModel(energy=energy, values=values)
template.plot(energy_range)
plt.grid(which="both")
new_model = template * PowerLawNormSpectralModel(norm=2, tilt=0)
print(new_model)
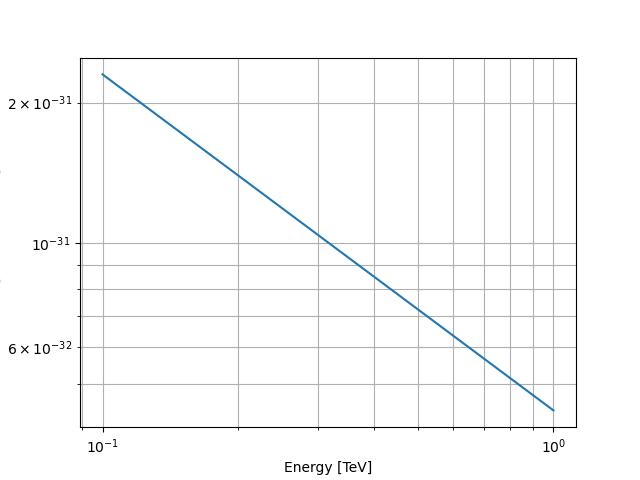
Out:
CompoundSpectralModel
Component 1 : TemplateSpectralModel
Component 2 : PowerLawNormSpectralModel
name value unit min max frozen error
--------- ---------- ---- --- --- ------ ---------
norm 2.0000e+00 nan nan False 0.000e+00
tilt 0.0000e+00 nan nan True 0.000e+00
reference 1.0000e+00 TeV nan nan True 0.000e+00
Operator : mul
YAML representation¶
Here is an example YAML file using the model:
Out:
components:
- name: template-model
type: SkyModel
spectral:
type: TemplateSpectralModel
energy:
data:
- 1000000.0
- 3000000.0
- 10000000.0
- 30000000.0
unit: MeV
values:
data:
- 4.4e-38
- 2.0e-38
- 8.8e-39
- 3.9e-39
unit: 1 / (cm2 MeV s)