Image processing and analysis tools (gammapy.image
)¶
Introduction¶
gammapy.image
contains data classes and methods for image based analysis
of gamma-ray data.
Getting Started¶
The central data structure in gammapy.image
is the SkyImage
class, which combines the raw data with WCS information, FITS I/O functionality
and many other methods, that allow easy handling, processing and plotting of
image based data. Here is a first example:
from gammapy.image import SkyImage
filename = '$GAMMAPY_EXTRA/datasets/fermi_2fhl/fermi_2fhl_vela.fits.gz'
image = SkyImage.read(filename, hdu=2)
image.show()
(Source code, png, hires.png, pdf)
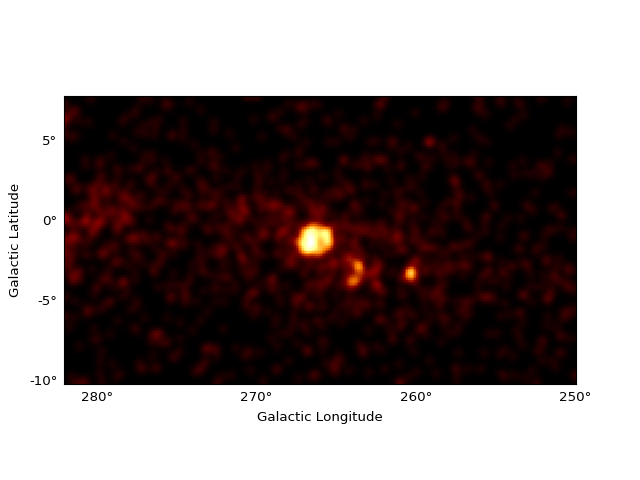
This loads a prepared Fermi 2FHL FITS image of the Vela region, creates a
SkyImage
and shows it on the the screen by calling SkyImage.show()
.
To explore further the SkyImage class try tab completion on the image
object
in an interactive python environment or see the Sky image page.
Using gammapy.image
¶
If you’d like to learn more about using gammapy.image
, read the following sub-pages:
Reference/API¶
gammapy.image Package¶
Sky images (2-dimensional: lon, lat).
Functions¶
asmooth_scales (n_scales[, factor, kernel]) |
Create list of Gaussian widths. |
block_reduce_hdu (input_hdu, block_size, func) |
Provides block reduce functionality for image HDUs. |
catalog_image (reference, psf[, catalog, ...]) |
Creates an image from a simulated catalog, or from 1FHL or 2FGL sources. |
catalog_table (catalog[, energy_bands]) |
Creates catalog table from published source catalog. |
colormap_hess ([transition, width]) |
Colormap often used in H.E.S.S. |
colormap_milagro ([transition, width, huestart]) |
Colormap often used in Milagro collaboration publications. |
fits_to_png (infile, outfile, draw[, dpi]) |
Plot FITS image in PNG format. |
fitsfigure_add_psf_inset (ff, psf, box[, ...]) |
Add PSF inset to FITSFigure instance. |
grayify_colormap (cmap[, mode]) |
Return a grayscale version a the colormap. |
illustrate_colormap (cmap, **kwargs) |
Illustrate color distribution and perceived luminance of a colormap. |
image_groupby (images, labels) |
Group pixel by labels. |
lon_lat_circle_mask (lons, lats, center_lon, ...) |
Produces a circular boolean mask array. |
lon_lat_rectangle_mask (lons, lats[, ...]) |
Produces a rectangular boolean mask array based on lat and lon limits. |
make_header ([nxpix, nypix, binsz, xref, ...]) |
Generate a FITS header from scratch. |
measure_containment (image, position, radius) |
Measure containment in a given circle around the source position. |
measure_containment_fraction (image, radius, ...) |
Measure containment fraction. |
measure_containment_radius (image, position) |
Measure containment radius of a source. |
measure_curve_of_growth (image, position[, ...]) |
Measure the curve of growth for a given source position. |
measure_image_moments (image) |
Compute 0th, 1st and 2nd moments of an image. |
measure_labeled_regions (data, labels[, tag, ...]) |
Measure source properties in image. |
process_image_pixels (images, kernel, out, ...) |
Process images for a given kernel and per-pixel function. |
radial_profile (image, center, radius) |
Image radial profile. |
radial_profile_label_image (image, center, radius) |
Image radial profile label image. |
Classes¶
ASmooth ([kernel, method, threshold, scales]) |
Adaptively smooth counts image. |
FermiLATBasicImageEstimator (reference, emin, ...) |
Estimate basic sky images for Fermi-LAT data. |
GalacticPlaneSurveyPanelPlot ([npanels, ...]) |
Plot Galactic plane survey images in multiple panels. |
IACTBasicImageEstimator (reference, emin, emax) |
Estimate the basic sky images for a set of IACT observations. |
ImageProfile (table) |
Image profile class. |
ImageProfileEstimator ([x_edges, method, axis]) |
Estimate profile from image. |
SkyImage ([name, data, wcs, unit, meta]) |
Sky image. |
SkyImageList ([images, meta]) |
List of SkyImage objects. |
gammapy.image.models Package¶
Morphology models.
Functions¶
gaussian_sum_moments (F, sigma, x, y, cov_matrix) |
Compute image moments with uncertainties for sum of Gaussians. |
make_test_model ([nsources, npix, ampl, ...]) |
Create a model of several Gaussian sources. |
read_ascii (filename, setter) |
Read from ASCII file. |
read_json (source, setter) |
Read from JSON file. |
write_all ([filename]) |
Dump source, fit results and conf results to a JSON file. |
write_ascii (pars, filename) |
Write to ASCII |
write_json (pars, filename) |
Write to JSON file. |
Classes¶
Delta2D (amplitude, x_0, y_0, **constraints) |
Two dimensional delta function . |
Gauss2DPDF ([sigma]) |
2D symmetric Gaussian PDF. |
ModelThetaCalculator (source, psf, fov, binsz) |
Compute containment radius for given radially symmetric source and psf as well as desired containment fraction. |
MorphModelImageCreator (cfg_file, exposure[, ...]) |
Create model images from a HGPS pipeline source config file. |
MultiGauss2D (sigmas[, norms]) |
Sum of multiple 2D Gaussians. |
Shell2D (amplitude, x_0, y_0, r_in[, width, ...]) |
Projected homogeneous radiating shell model. |
Sphere2D (amplitude, x_0, y_0, r_0[, normed]) |
Projected homogeneous radiating sphere model. |
ThetaCalculator (dist, theta_max, n_bins[, ...]) |
Provides methods containment_fraction(theta) and containment_radius(containment_fraction) given some 1D distribution (not necessarily normalized). |
ThetaCalculator2D (dist, x, y) |
Methods to compute theta and containment for a given 2D probability distribution image. |
ThetaCalculatorScipy (dist, theta_max[, ...]) |
Same functionality as NumericalThetaCalculator, but uses scipy.integrate.quad and scipy.optimize.fsolve instead. |