Note
Click here to download the full example code
Absorbed Spectral Model¶
This model evaluates absorbed spectral model.
The spectral model is evaluated, and then multiplied with an EBL absorption factor given by
\[\exp{ \left ( -\alpha \times \tau(E, z) \right )}\]
where \(\tau(E, z)\) is the optical depth predicted by the model
(Absorption
), which depends on the energy of the gamma-rays and the
redshift z of the source, and \(\alpha\) is a scale factor
(default: 1) for the optical depth.
Example plot¶
Here is an example plot of the model:
from astropy import units as u
import matplotlib.pyplot as plt
from gammapy.modeling.models import (
AbsorbedSpectralModel,
Absorption,
Models,
PowerLawSpectralModel,
SkyModel,
)
redshift = 0.117
absorption = Absorption.read_builtin("dominguez")
# Spectral model corresponding to PKS 2155-304 (quiescent state)
index = 3.53
amplitude = 1.81 * 1e-12 * u.Unit("cm-2 s-1 TeV-1")
reference = 1 * u.TeV
pwl = PowerLawSpectralModel(index=index, amplitude=amplitude, reference=reference)
# EBL + PWL model
model = AbsorbedSpectralModel(
spectral_model=pwl, absorption=absorption, parameter=redshift
)
energy_range = [0.1, 100] * u.TeV
model.plot(energy_range)
plt.grid(which="both")
plt.ylim(1e-24, 1e-8)
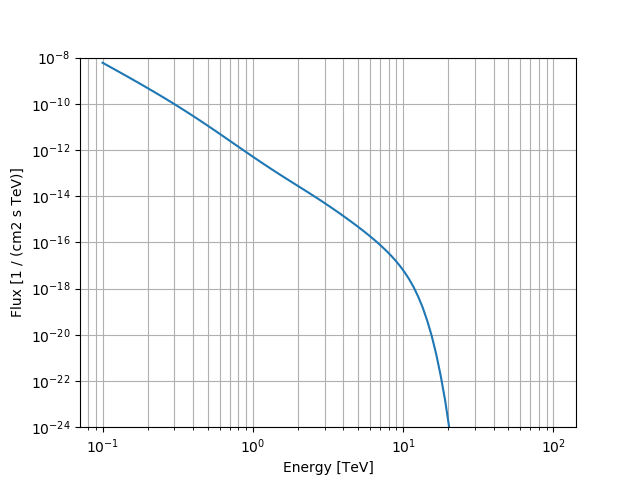
YAML representation¶
Here is an example YAML file using the model:
Out:
components:
- name: absorbed-model
type: SkyModel
spectral:
type: AbsorbedSpectralModel
base_model:
type: PowerLawSpectralModel
parameters:
- {name: index, value: 3.53, unit: '', min: .nan, max: .nan, frozen: false}
- {name: amplitude, value: 1.81e-12, unit: cm-2 s-1 TeV-1, min: .nan,
max: .nan, frozen: false}
- {name: reference, value: 1.0, unit: TeV, min: .nan, max: .nan, frozen: true}
absorption:
type: Absorption
filename: !!python/object/apply:pathlib.PosixPath [/, Users, adonath,
data, gammapy-data, ebl, ebl_dominguez11.fits.gz]
absorption_parameter: {name: redshift, value: 0.117}
parameters:
- {name: redshift, value: 0.117, unit: '', min: 0.009999999776482582, max: 3.990000009536743,
frozen: true}
- {name: alpha_norm, value: 1.0, unit: '', min: .nan, max: .nan, frozen: true}