detect - Source detection¶
Introduction¶
The gammapy.detect
submodule includes low level functions to compute
significance and test statistics images as well as some high level source
detection method prototypes.
Detailed description of the methods can be found in [Stewart2009] and [LiMa1983].
Note that in Gammapy maps are stored as Numpy arrays, which implies that it’s very easy to use scikit-image or photutils or other packages that have advanced image analysis and source detection methods readily available.
Computation of TS images¶
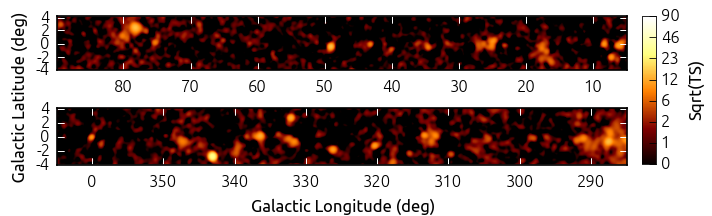
Test statistics image computed using TSMapEstimator
for an
example Fermi dataset.
The gammapy.detect
module includes a high performance
TSMapEstimator
class to compute test statistics (TS) images
for gamma-ray data. The implementation is based on the method described
in [Stewart2009].
Assuming a certain source morphology, which can be defined by any
astropy.convolution.Kernel2D
instance, the amplitude of the morphology model
is fitted at every pixel of the input data using a Poisson maximum likelihood
procedure. As input data a counts, background and exposure images have to be
provided. Based on the best fit flux amplitude, the change in TS, compared to
the null hypothesis is computed using cash
statistics.
To optimize the performance of the code, the fitting procedure is simplified by finding roots of the derivative of the fit statistics with respect to the flux amplitude. This approach is described in detail in Appendix A of [Stewart2009].
The following example shows how to compute a TS image for Fermi-LAT 3FHL galactic center data:
from astropy.convolution import Gaussian2DKernel
from gammapy.detect import TSMapEstimator
from gammapy.maps import Map
from gammapy.cube import PSFKernel
counts = Map.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-counts.fits.gz")
background = Map.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-background.fits.gz")
exposure = Map.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-exposure.fits.gz")
maps = {
"counts": counts,
"background": background,
"exposure": exposure,
}
kernel = PSFKernel.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-psf.fits.gz")
ts_estimator = TSMapEstimator()
result = ts_estimator.run(maps, kernel.data)
The function returns an dictionary, that bundles all resulting maps. E.g. here’s how to find the largest TS value:
import numpy as np
np.nanmax(result['ts'].data)
Computation of Li & Ma significance images¶
The method derived by [LiMa1983] is one of the standard methods to determine
detection significances for gamma-ray sources. Using the same prepared Fermi
dataset as above, the corresponding images can be computed using the
compute_lima_image
function:
from astropy.convolution import Tophat2DKernel
from gammapy.maps import Map
from gammapy.detect import compute_lima_image
counts = Map.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-counts.fits.gz")
background = Map.read("$GAMMAPY_DATA/fermi-3fhl-gc/fermi-3fhl-gc-background.fits.gz")
kernel = Tophat2DKernel(5)
result = compute_lima_image(counts, background, kernel)
The function returns a dictionary, that bundles all resulting images such as significance, flux and correlated counts and excess images.
Reference/API¶
gammapy.detect Package¶
Source detection and measurement methods.
Functions¶
|
Compute Li & Ma significance and flux images for known background. |
|
Compute Li & Ma significance and flux images for on-off observations. |
|
Find local peaks in an image. |
Classes¶
|
Adaptively smooth counts image. |
|
Estimate background and exclusion mask iteratively. |
|
Compute TS map from a MapDataset using different optimization methods. |