Note
Click here to download the full example code
Compound Spectral Model¶
This model is formed by the arithmetic combination of any two other spectral models.
Example plot¶
Here is an example plot of the model:
import operator
from astropy import units as u
import matplotlib.pyplot as plt
from gammapy.modeling.models import (
CompoundSpectralModel,
LogParabolaSpectralModel,
Models,
PowerLawSpectralModel,
SkyModel,
)
energy_range = [0.1, 100] * u.TeV
pwl = PowerLawSpectralModel(
index=2.0, amplitude="1e-12 cm-2 s-1 TeV-1", reference="1 TeV"
)
lp = LogParabolaSpectralModel(
amplitude="1e-12 cm-2 s-1 TeV-1", reference="10 TeV", alpha=2.0, beta=1.0
)
model = CompoundSpectralModel(pwl, lp, operator.add)
model.plot(energy_range)
plt.grid(which="both")
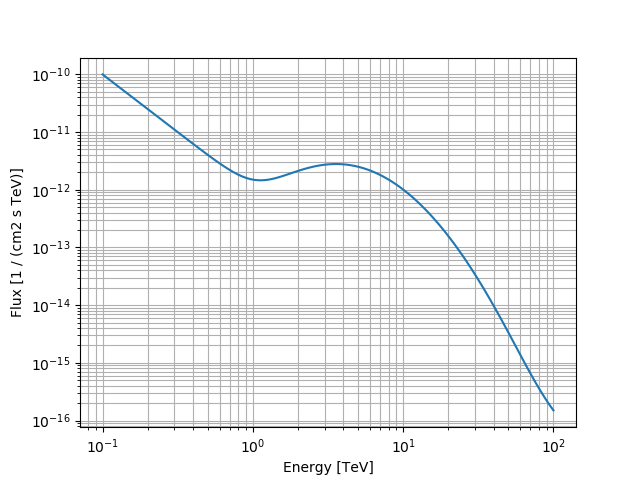
YAML representation¶
Here is an example YAML file using the model:
Out:
components:
- name: compound-model
type: SkyModel
spectral:
model1:
type: PowerLawSpectralModel
parameters:
- {name: index, value: 2.0, unit: '', min: .nan, max: .nan, frozen: false,
error: 0}
- {name: amplitude, value: 1.0e-12, unit: cm-2 s-1 TeV-1, min: .nan, max: .nan,
frozen: false, error: 0}
- {name: reference, value: 1.0, unit: TeV, min: .nan, max: .nan, frozen: true,
error: 0}
model2:
type: LogParabolaSpectralModel
parameters:
- {name: amplitude, value: 1.0e-12, unit: cm-2 s-1 TeV-1, min: .nan, max: .nan,
frozen: false, error: 0}
- {name: reference, value: 10.0, unit: TeV, min: .nan, max: .nan, frozen: true,
error: 0}
- {name: alpha, value: 2.0, unit: '', min: .nan, max: .nan, frozen: false,
error: 0}
- {name: beta, value: 1.0, unit: '', min: .nan, max: .nan, frozen: false,
error: 0}
operator: !!python/name:_operator.add ''