This is a fixed-text formatted version of a Jupyter notebook
You may download all the notebooks in the documentation as a tar file.
Source files: fitting.ipynb | fitting.py
Fitting¶
Prerequisites¶
Knowledge of spectral analysis to produce 1D On-Off datasets, see the following tutorial
Reading of pre-computed datasets see the MWL tutorial
General knowledge on statistics and optimization methods
Proposed approach¶
This is a hands-on tutorial to gammapy.modeling
, showing how to do perform a Fit in gammapy. The emphasis here is on interfacing the Fit
class and inspecting the errors. To see an analysis example of how datasets and models interact, see the model management notebook. As an example, in this notebook, we are going to work with HESS data of the Crab Nebula and show in particular how to : - perform a spectral analysis - use different fitting backends - access
covariance matrix information and parameter errors - compute likelihood profile - compute confidence contours
See also: Models gallery tutorial and docs/modeling/index.rst
.
The setup¶
[1]:
import numpy as np
from astropy import units as u
import matplotlib.pyplot as plt
from matplotlib.ticker import StrMethodFormatter
import scipy.stats as st
from gammapy.modeling import Fit
from gammapy.datasets import Datasets, SpectrumDatasetOnOff
from gammapy.modeling.models import LogParabolaSpectralModel, SkyModel
from gammapy.visualization.utils import plot_contour_line
from itertools import combinations
Model and dataset¶
First we define the source model, here we need only a spectral model for which we choose a log-parabola
[2]:
crab_spectrum = LogParabolaSpectralModel(
amplitude=1e-11 / u.cm ** 2 / u.s / u.TeV,
reference=1 * u.TeV,
alpha=2.3,
beta=0.2,
)
crab_spectrum.alpha.max = 3
crab_spectrum.alpha.min = 1
crab_model = SkyModel(spectral_model=crab_spectrum, name="crab")
The data and background are read from pre-computed ON/OFF datasets of HESS observations, for simplicity we stack them together. Then we set the model and fit range to the resulting dataset.
[3]:
datasets = []
for obs_id in [23523, 23526]:
dataset = SpectrumDatasetOnOff.read(
f"$GAMMAPY_DATA/joint-crab/spectra/hess/pha_obs{obs_id}.fits"
)
datasets.append(dataset)
dataset_hess = Datasets(datasets).stack_reduce(name="HESS")
# Set model and fit range
dataset_hess.models = crab_model
e_min = 0.66 * u.TeV
e_max = 30 * u.TeV
dataset_hess.mask_fit = dataset_hess.counts.geom.energy_mask(e_min, e_max)
Fitting options¶
First let’s create a Fit
instance:
[4]:
scipy_opts = {
"method": "L-BFGS-B",
"options": {"ftol": 1e-4, "gtol": 1e-05},
"backend": "scipy",
}
fit_scipy = Fit(store_trace=True, optimize_opts=scipy_opts)
By default the fit is performed using MINUIT, you can select alternative optimizers and set their option using the optimize_opts
argument of the Fit.run()
method. In addition we have specified to store the trace of parameter values of the fit.
Note that, for now, covaraince matrix and errors are computed only for the fitting with MINUIT. However depending on the problem other optimizers can better perform, so sometimes it can be useful to run a pre-fit with alternative optimization methods.
[5]:
%%time
result_scipy = fit_scipy.run([dataset_hess])
CPU times: user 116 ms, sys: 3.69 ms, total: 120 ms
Wall time: 119 ms
[6]:
%%time
sherpa_opts = {"method": "simplex", "ftol": 1e-3, "maxfev": int(1e4)}
fit_sherpa = Fit(store_trace=True, backend="sherpa", optimize_opts=sherpa_opts)
results_simplex = fit_sherpa.run([dataset_hess])
No covariance estimate - not supported by this backend.
CPU times: user 188 ms, sys: 3.23 ms, total: 191 ms
Wall time: 355 ms
For the “minuit” backend see https://iminuit.readthedocs.io/en/latest/reference.html for a detailed description of the available options. If there is an entry ‘migrad_opts’, those options will be passed to iminuit.Minuit.migrad. Additionally you can set the fit tolerance using the tol option. The minimization will stop when the estimated distance to the minimum is less than 0.001*tol (by default tol=0.1). The strategy option change the speed and accuracy of the optimizer: 0 fast, 1 default, 2 slow but accurate. If you want more reliable error estimates, you should run the final fit with strategy 2.
[7]:
%%time
fit = Fit(store_trace=True)
minuit_opts = {"tol": 0.001, "strategy": 1}
fit.backend = "minuit"
fit.optimize_opts = minuit_opts
result_minuit = fit.run([dataset_hess])
CPU times: user 44.7 ms, sys: 1.78 ms, total: 46.5 ms
Wall time: 45.1 ms
Fit quality assessment¶
There are various ways to check the convergence and quality of a fit. Among them:
Refer to the automatically-generated results dictionary:
[8]:
print(result_scipy)
OptimizeResult
backend : scipy
method : L-BFGS-B
success : True
message : CONVERGENCE: REL_REDUCTION_OF_F_<=_FACTR*EPSMCH
nfev : 60
total stat : 30.35
OptimizeResult
backend : scipy
method : L-BFGS-B
success : True
message : CONVERGENCE: REL_REDUCTION_OF_F_<=_FACTR*EPSMCH
nfev : 60
total stat : 30.35
[9]:
print(results_simplex)
OptimizeResult
backend : sherpa
method : simplex
success : True
message : Optimization terminated successfully
nfev : 135
total stat : 30.35
[10]:
print(result_minuit)
OptimizeResult
backend : minuit
method : migrad
success : True
message : Optimization terminated successfully.
nfev : 37
total stat : 30.35
OptimizeResult
backend : minuit
method : migrad
success : True
message : Optimization terminated successfully.
nfev : 37
total stat : 30.35
Check the trace of the fit e.g. in case the fit did not converge properly
[11]:
result_minuit.trace
[11]:
total_stat | crab.spectral.amplitude | crab.spectral.alpha | crab.spectral.beta |
---|---|---|---|
float64 | float64 | float64 | float64 |
30.349530550400765 | 3.812242568529405e-11 | 2.1957469269201617 | 0.22648272085464238 |
30.34972457253742 | 3.815793736236919e-11 | 2.1957469269201617 | 0.22648272085464238 |
30.34971173244832 | 3.8086914008218915e-11 | 2.1957469269201617 | 0.22648272085464238 |
30.349539326752044 | 3.812951391706827e-11 | 2.1957469269201617 | 0.22648272085464238 |
30.349536722665142 | 3.811533745351983e-11 | 2.1957469269201617 | 0.22648272085464238 |
30.35050886992802 | 3.812242568529405e-11 | 2.198424618760706 | 0.22648272085464238 |
30.350543934671048 | 3.812242568529405e-11 | 2.193067774847982 | 0.22648272085464238 |
30.34953899182041 | 3.812242568529405e-11 | 2.196014762144314 | 0.22648272085464238 |
30.349542025993827 | 3.812242568529405e-11 | 2.1954790770936845 | 0.22648272085464238 |
30.35029517198719 | 3.812242568529405e-11 | 2.1957469269201617 | 0.22788952829627426 |
30.35033282113968 | 3.812242568529405e-11 | 2.1957469269201617 | 0.22507591341301048 |
30.349536744191 | 3.812242568529405e-11 | 2.1957469269201617 | 0.22662340159880556 |
30.34954002542583 | 3.812242568529405e-11 | 2.1957469269201617 | 0.22634204011047918 |
30.349530632960807 | 3.812180829128438e-11 | 2.195767328548783 | 0.22649745095864532 |
30.349530467417637 | 3.81221697206664e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349537982307382 | 3.8129257953704704e-11 | 2.195755385216711 | 0.22648882779045226 |
30.34953790131041 | 3.8115081487628087e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349538145487614 | 3.81221697206664e-11 | 2.195987422083998 | 0.22648882779045226 |
30.349537737092085 | 3.81221697206664e-11 | 2.195523337389243 | 0.22648882779045226 |
30.349537804377285 | 3.81221697206664e-11 | 2.195755385216711 | 0.2266262374110642 |
30.349538077967896 | 3.81221697206664e-11 | 2.195755385216711 | 0.22635141816984033 |
30.349530467417637 | 3.81221697206664e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349537982307382 | 3.8129257953704704e-11 | 2.195755385216711 | 0.22648882779045226 |
30.34953790131041 | 3.8115081487628087e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349538145487614 | 3.81221697206664e-11 | 2.195987422083998 | 0.22648882779045226 |
30.349537737092085 | 3.81221697206664e-11 | 2.195523337389243 | 0.22648882779045226 |
30.349537804377285 | 3.81221697206664e-11 | 2.195755385216711 | 0.2266262374110642 |
30.349538077967896 | 3.81221697206664e-11 | 2.195755385216711 | 0.22635141816984033 |
30.34953077465702 | 3.8123587367274056e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349530758129603 | 3.8120752074058735e-11 | 2.195755385216711 | 0.22648882779045226 |
30.349530807510003 | 3.81221697206664e-11 | 2.195801793467399 | 0.22648882779045226 |
30.349530725235304 | 3.81221697206664e-11 | 2.195708976527616 | 0.22648882779045226 |
30.34953073944574 | 3.81221697206664e-11 | 2.195755385216711 | 0.22651630971457465 |
30.3495307932899 | 3.81221697206664e-11 | 2.195755385216711 | 0.22646134586632988 |
30.34953581489015 | 3.8129257953704704e-11 | 2.195987422083998 | 0.22648882779045226 |
30.34953715865348 | 3.8129257953704704e-11 | 2.195755385216711 | 0.2266262374110642 |
30.349559366841248 | 3.81221697206664e-11 | 2.195987422083998 | 0.2266262374110642 |
Check that the fitted values and errors for all parameters are reasonable, and no fitted parameter value is “too close” - or even outside - its allowed min-max range
[12]:
result_minuit.parameters.to_table()
[12]:
type | name | value | unit | error | min | max | frozen | link |
---|---|---|---|---|---|---|---|---|
str8 | str9 | float64 | str14 | float64 | float64 | float64 | bool | str1 |
spectral | amplitude | 3.8122e-11 | cm-2 s-1 TeV-1 | 3.546e-12 | nan | nan | False | |
spectral | reference | 1.0000e+00 | TeV | 0.000e+00 | nan | nan | True | |
spectral | alpha | 2.1958e+00 | 2.626e-01 | 1.000e+00 | 3.000e+00 | False | ||
spectral | beta | 2.2649e-01 | 1.397e-01 | nan | nan | False |
Plot fit statistic profiles for all fitted parameters, using gammapy.modeling.Fit.stat_profile()
. For a good fit and error estimate each profile should be parabolic. The specification for each fit statistic profile can be changed on the gammapy.modeling.Parameter
object, which has .scan_min
, .scan_max
, .scan_n_values
and .scan_n_sigma
attributes.
[13]:
total_stat = result_minuit.total_stat
fig, axes = plt.subplots(nrows=1, ncols=3, figsize=(14, 4))
for ax, par in zip(axes, crab_model.parameters.free_parameters):
par.scan_n_values = 17
profile = fit.stat_profile(datasets=[dataset_hess], parameter=par)
ax.plot(profile[f"{par.name}_scan"], profile["stat_scan"] - total_stat)
ax.set_xlabel(f"{par.unit}")
ax.set_ylabel("Delta TS")
ax.set_title(f"{par.name}: {par.value:.1e} +- {par.error:.1e}")
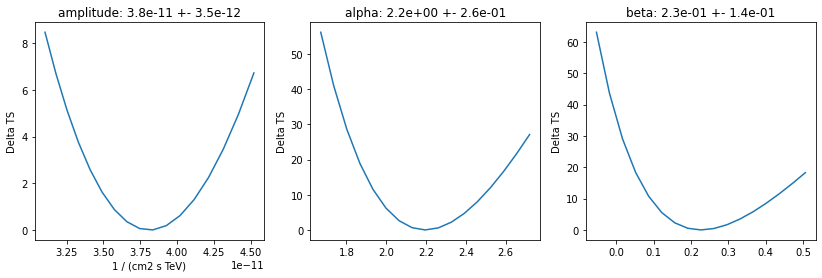
Inspect model residuals. Those can always be accessed using ~Dataset.residuals()
, that will return an array in case a the fitted Dataset
is a SpectrumDataset
and a full cube in case of a MapDataset
. For more details, we refer here to the dedicated fitting tutorials: analysis_3d.ipynb (for MapDataset
fitting) and spectrum_analysis.ipynb (for SpectrumDataset
fitting).
Covariance and parameters errors¶
After the fit the covariance matrix is attached to the model. You can get the error on a specific parameter by accessing the .error
attribute:
[14]:
crab_model.spectral_model.alpha.error
[14]:
0.2625817875684573
And you can plot the total parameter correlation as well:
[15]:
crab_model.covariance.plot_correlation()
[15]:
<AxesSubplot:>
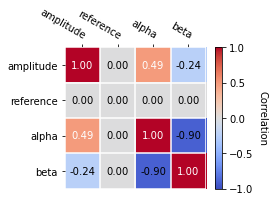
As an example, this step is needed to produce a butterfly plot showing the envelope of the model taking into account parameter uncertainties.
[16]:
energy_bounds = [1, 10] * u.TeV
crab_spectrum.plot(energy_bounds=energy_bounds, energy_power=2)
ax = crab_spectrum.plot_error(energy_bounds=energy_bounds, energy_power=2)
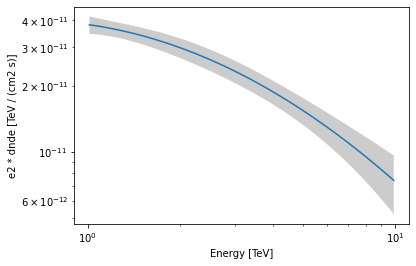
Confidence contours¶
In most studies, one wishes to estimate parameters distribution using observed sample data. A 1-dimensional confidence interval gives an estimated range of values which is likely to include an unknown parameter. A confidence contour is a 2-dimensional generalization of a confidence interval, often represented as an ellipsoid around the best-fit value.
Gammapy offers two ways of computing confidence contours, in the dedicated methods Fit.minos_contour()
and Fit.stat_profile()
. In the following sections we will describe them.
An important point to keep in mind is: what does a :math:`Nsigma` confidence contour really mean? The answer is it represents the points of the parameter space for which the model likelihood is \(N\sigma\) above the minimum. But one always has to keep in mind that 1 standard deviation in two dimensions has a smaller coverage probability than 68%, and similarly for all other levels. In particular, in 2-dimensions the probability enclosed by the \(N\sigma\) confidence contour is \(P(N)=1-e^{-N^2/2}\).
Computing contours using Fit.stat_contour()
¶
After the fit, MINUIT offers the possibility to compute the confidence confours. gammapy provides an interface to this functionality through the Fit
object using the .stat_contour
method. Here we defined a function to automate the contour production for the different parameterer and confidence levels (expressed in term of sigma):
[17]:
def make_contours(fit, datasets, result, npoints, sigmas):
cts_sigma = []
for sigma in sigmas:
contours = dict()
for par_1, par_2 in combinations(["alpha", "beta", "amplitude"], r=2):
contour = fit.stat_contour(
datasets=datasets,
x=result.parameters[par_1],
y=result.parameters[par_2],
numpoints=npoints,
sigma=sigma,
)
contours[f"contour_{par_1}_{par_2}"] = {
par_1: contour[par_1].tolist(),
par_2: contour[par_2].tolist(),
}
cts_sigma.append(contours)
return cts_sigma
Now we can compute few contours.
[18]:
%%time
sigmas = [1, 2]
cts_sigma = make_contours(
fit=fit,
datasets=[dataset_hess],
result=result_minuit,
npoints=10,
sigmas=sigmas,
)
CPU times: user 4.49 s, sys: 19.1 ms, total: 4.51 s
Wall time: 4.51 s
Then we prepare some aliases and annotations in order to make the plotting nicer.
[19]:
pars = {
"phi": r"$\phi_0 \,/\,(10^{-11}\,{\rm TeV}^{-1} \, {\rm cm}^{-2} {\rm s}^{-1})$",
"alpha": r"$\alpha$",
"beta": r"$\beta$",
}
panels = [
{
"x": "alpha",
"y": "phi",
"cx": (lambda ct: ct["contour_alpha_amplitude"]["alpha"]),
"cy": (
lambda ct: np.array(1e11)
* ct["contour_alpha_amplitude"]["amplitude"]
),
},
{
"x": "beta",
"y": "phi",
"cx": (lambda ct: ct["contour_beta_amplitude"]["beta"]),
"cy": (
lambda ct: np.array(1e11)
* ct["contour_beta_amplitude"]["amplitude"]
),
},
{
"x": "alpha",
"y": "beta",
"cx": (lambda ct: ct["contour_alpha_beta"]["alpha"]),
"cy": (lambda ct: ct["contour_alpha_beta"]["beta"]),
},
]
Finally we produce the confidence contours figures.
[20]:
fig, axes = plt.subplots(1, 3, figsize=(16, 5))
colors = ["m", "b", "c"]
for p, ax in zip(panels, axes):
xlabel = pars[p["x"]]
ylabel = pars[p["y"]]
for ks in range(len(cts_sigma)):
plot_contour_line(
ax,
p["cx"](cts_sigma[ks]),
p["cy"](cts_sigma[ks]),
lw=2.5,
color=colors[ks],
label=f"{sigmas[ks]}" + r"$\sigma$",
)
ax.set_xlabel(xlabel)
ax.set_ylabel(ylabel)
plt.legend()
plt.tight_layout()
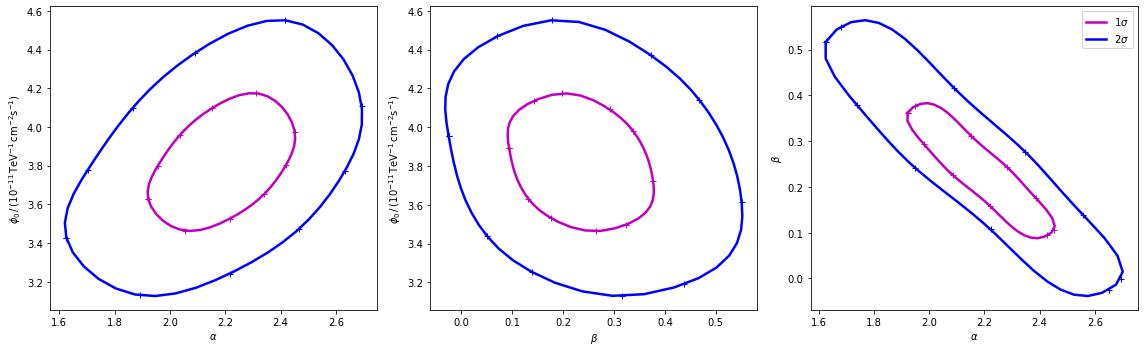
Computing contours using Fit.stat_surface()
¶
This alternative method for the computation of confidence contours, although more time consuming than Fit.minos_contour()
, is expected to be more stable. It consists of a generalization of Fit.stat_profile()
to a 2-dimensional parameter space. The algorithm is very simple: - First, passing two arrays of parameters values, a 2-dimensional discrete parameter space is defined; - For each node of the parameter space, the two parameters of interest are frozen. This way, a likelihood value
(\(-2\mathrm{ln}\,\mathcal{L}\), actually) is computed, by either freezing (default) or fitting all nuisance parameters; - Finally, a 2-dimensional surface of \(-2\mathrm{ln}(\mathcal{L})\) values is returned. Using that surface, one can easily compute a surface of \(TS = -2\Delta\mathrm{ln}(\mathcal{L})\) and compute confidence contours.
Let’s see it step by step.
First of all, we can notice that this method is “backend-agnostic”, meaning that it can be run with MINUIT, sherpa or scipy as fitting tools. Here we will stick with MINUIT, which is the default choice:
[ ]:
As an example, we can compute the confidence contour for the alpha
and beta
parameters of the dataset_hess
. Here we define the parameter space:
[21]:
result = result_minuit
par_alpha = result.parameters["alpha"]
par_beta = result.parameters["beta"]
par_alpha.scan_values = np.linspace(1.55, 2.7, 20)
par_beta.scan_values = np.linspace(-0.05, 0.55, 20)
Then we run the algorithm, by choosing reoptimize=False
for the sake of time saving. In real life applications, we strongly recommend to use reoptimize=True
, so that all free nuisance parameters will be fit at each grid node. This is the correct way, statistically speaking, of computing confidence contours, but is expected to be time consuming.
[22]:
fit = Fit(backend="minuit", optimize_opts={"print_level": 0})
stat_surface = fit.stat_surface(
datasets=[dataset_hess],
x=par_alpha,
y=par_beta,
reoptimize=False,
)
In order to easily inspect the results, we can convert the \(-2\mathrm{ln}(\mathcal{L})\) surface to a surface of statistical significance (in units of Gaussian standard deviations from the surface minimum):
[23]:
# Compute TS
TS = stat_surface["stat_scan"] - result.total_stat
[24]:
# Compute the corresponding statistical significance surface
stat_surface = np.sqrt(TS.T)
Notice that, as explained before, \(1\sigma\) contour obtained this way will not contain 68% of the probability, but rather
[25]:
# Compute the corresponding statistical significance surface
# p_value = 1 - st.chi2(df=1).cdf(TS)
# gaussian_sigmas = st.norm.isf(p_value / 2).T
Finally, we can plot the surface values together with contours:
[26]:
fig, ax = plt.subplots(figsize=(8, 6))
x_values = par_alpha.scan_values
y_values = par_beta.scan_values
# plot surface
im = ax.pcolormesh(x_values, y_values, stat_surface, shading="auto")
fig.colorbar(im, label="sqrt(TS)")
ax.set_xlabel(f"{par_alpha.name}")
ax.set_ylabel(f"{par_beta.name}")
# We choose to plot 1 and 2 sigma confidence contours
levels = [1, 2]
contours = ax.contour(
x_values, y_values, stat_surface, levels=levels, colors="white"
)
ax.clabel(contours, fmt="%.0f$\,\sigma$", inline=3, fontsize=15);
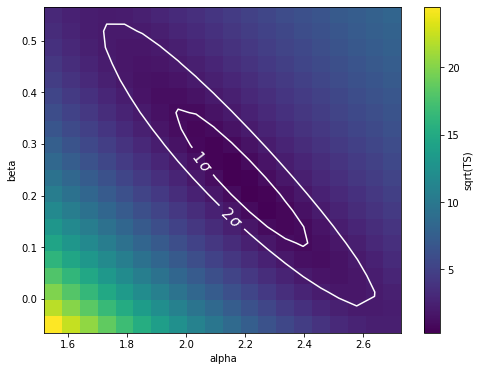
Note that, if computed with reoptimize=True
, this plot would be completely consistent with the third panel of the plot produced with Fit.stat_contour
(try!).
Finally, it is always remember that confidence contours are approximations. In particular, when the parameter range boundaries are close to the contours lines, it is expected that the statistical meaning of the contours is not well defined. That’s why we advise to always choose a parameter space that com contain the contours you’re interested in.
[ ]: