This is a fixed-text formatted version of a Jupyter notebook
You may download all the notebooks in the documentation as a tar file.
Source files: light_curve.ipynb | light_curve.py
Light curves¶
Prerequisites¶
Knowledge of the high level interface to perform data reduction, see first gammapy analysis with the high level interface tutorial
Context¶
This tutorial presents how light curve extraction is performed in gammapy, i.e. how to measure the flux of a source in different time bins.
Cherenkov telescopes usually work with observing runs and distribute data according to this basic time interval. A typical use case is to look for variability of a source on various time binnings: observation run-wise binning, nightly, weekly etc.
Objective: The Crab nebula is not known to be variable at TeV energies, so we expect constant brightness within statistical and systematic errors. Compute per-observation and nightly fluxes of the four Crab nebula observations from the H.E.S.S. first public test data releaseoto check it.
Proposed approach¶
We will demonstrate how to compute a gammapy.estimators.LightCurve
from 3D reduced datasets (gammapy.datasets.MapDataset
) as well as 1D ON-OFF spectral datasets (gammapy.datasets.SpectrumDatasetOnOff
).
The data reduction will be performed with the high level interface for the data reduction. Then we will use the gammapy.estimators.LightCurveEstimator
class, which is able to extract a light curve independently of the dataset type.
Setup¶
As usual, we’ll start with some general imports…
[1]:
%matplotlib inline
import matplotlib.pyplot as plt
import astropy.units as u
from astropy.coordinates import SkyCoord
import logging
from astropy.time import Time
log = logging.getLogger(__name__)
Now let’s import gammapy specific classes and functions
[2]:
from gammapy.modeling.models import PowerLawSpectralModel
from gammapy.modeling.models import PointSpatialModel
from gammapy.modeling.models import SkyModel, Models
from gammapy.modeling import Fit
from gammapy.estimators import LightCurveEstimator
from gammapy.analysis import Analysis, AnalysisConfig
Analysis configuration¶
For the 1D and 3D extraction, we will use the same CrabNebula configuration than in the notebook analysis_1.ipynb using the high level interface of Gammapy.
From the high level interface, the data reduction for those observations is performed as followed
Building the 3D analysis configuration¶
[3]:
conf_3d = AnalysisConfig()
Definition of the data selection¶
Here we use the Crab runs from the HESS DL3 data release 1
[4]:
conf_3d.observations.obs_ids = [23523, 23526, 23559, 23592]
Definition of the dataset geometry¶
[5]:
# We want a 3D analysis
conf_3d.datasets.type = "3d"
# We want to extract the data by observation and therefore to not stack them
conf_3d.datasets.stack = False
# Here is the WCS geometry of the Maps
conf_3d.datasets.geom.wcs.skydir = dict(
frame="icrs", lon=83.63308 * u.deg, lat=22.01450 * u.deg
)
conf_3d.datasets.geom.wcs.binsize = 0.02 * u.deg
conf_3d.datasets.geom.wcs.width = dict(width=1 * u.deg, height=1 * u.deg)
# We define a value for the IRF Maps binsize
conf_3d.datasets.geom.wcs.binsize_irf = 0.2 * u.deg
# Define energy binning for the Maps
conf_3d.datasets.geom.axes.energy = dict(
min=0.7 * u.TeV, max=10 * u.TeV, nbins=5
)
conf_3d.datasets.geom.axes.energy_true = dict(
min=0.3 * u.TeV, max=20 * u.TeV, nbins=20
)
Run the 3D data reduction¶
[6]:
analysis_3d = Analysis(conf_3d)
analysis_3d.get_observations()
analysis_3d.get_datasets()
Setting logging config: {'level': 'INFO', 'filename': None, 'filemode': None, 'format': None, 'datefmt': None}
Fetching observations.
No HDU found matching: OBS_ID = 23523, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23526, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23559, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23592, HDU_TYPE = rad_max, HDU_CLASS = None
Number of selected observations: 4
Creating reference dataset and makers.
Creating the background Maker.
No background maker set. Check configuration.
Start the data reduction loop.
Define the model to be used¶
Here we don’t try to fit the model parameters to the whole dataset, but we use predefined values instead.
[7]:
target_position = SkyCoord(ra=83.63308, dec=22.01450, unit="deg")
spatial_model = PointSpatialModel(
lon_0=target_position.ra, lat_0=target_position.dec, frame="icrs"
)
spectral_model = PowerLawSpectralModel(
index=2.702,
amplitude=4.712e-11 * u.Unit("1 / (cm2 s TeV)"),
reference=1 * u.TeV,
)
sky_model = SkyModel(
spatial_model=spatial_model, spectral_model=spectral_model, name="crab"
)
# Now we freeze these parameters that we don't want the light curve estimator to change
sky_model.parameters["index"].frozen = True
sky_model.parameters["lon_0"].frozen = True
sky_model.parameters["lat_0"].frozen = True
We assign them the model to be fitted to each dataset
[8]:
models = Models([sky_model])
analysis_3d.set_models(models)
Reading model.
Models
Component 0: SkyModel
Name : crab
Datasets names : None
Spectral model type : PowerLawSpectralModel
Spatial model type : PointSpatialModel
Temporal model type :
Parameters:
index (frozen) : 2.702
amplitude : 4.71e-11 +/- 0.0e+00 1 / (cm2 s TeV)
reference (frozen) : 1.000 TeV
lon_0 (frozen) : 83.633 deg
lat_0 (frozen) : 22.015 deg
Component 1: FoVBackgroundModel
Name : 38q7tq0c-bkg
Datasets names : ['38q7tq0c']
Spectral model type : PowerLawNormSpectralModel
Parameters:
norm : 1.000 +/- 0.00
tilt (frozen) : 0.000
reference (frozen) : 1.000 TeV
Component 2: FoVBackgroundModel
Name : 4nDYkSIj-bkg
Datasets names : ['4nDYkSIj']
Spectral model type : PowerLawNormSpectralModel
Parameters:
norm : 1.000 +/- 0.00
tilt (frozen) : 0.000
reference (frozen) : 1.000 TeV
Component 3: FoVBackgroundModel
Name : eXBkaW8t-bkg
Datasets names : ['eXBkaW8t']
Spectral model type : PowerLawNormSpectralModel
Parameters:
norm : 1.000 +/- 0.00
tilt (frozen) : 0.000
reference (frozen) : 1.000 TeV
Component 4: FoVBackgroundModel
Name : 2Guq4XT9-bkg
Datasets names : ['2Guq4XT9']
Spectral model type : PowerLawNormSpectralModel
Parameters:
norm : 1.000 +/- 0.00
tilt (frozen) : 0.000
reference (frozen) : 1.000 TeV
Light Curve estimation: by observation¶
We can now create the light curve estimator.
We pass it the list of datasets and the name of the model component for which we want to build the light curve. We can optionally ask for parameters reoptimization during fit, that is most of the time to fit background normalization in each time bin.
If we don’t set any time interval, the gammapy.estimators.LightCurveEstimator
is determines the flux of each dataset and places it at the corresponding time in the light curve. Here one dataset equals to one observing run.
[9]:
lc_maker_3d = LightCurveEstimator(
energy_edges=[1, 10] * u.TeV, source="crab", reoptimize=False
)
lc_3d = lc_maker_3d.run(analysis_3d.datasets)
The LightCurve object contains a table which we can explore.
[10]:
lc_3d.plot(axis_name="time")
[10]:
<AxesSubplot:xlabel='Time [iso]', ylabel='dnde (1 / (cm2 s TeV))'>
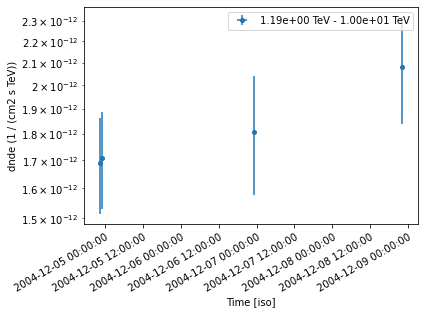
[11]:
table = lc_3d.to_table(format="lightcurve", sed_type="flux")
table["time_min", "time_max", "e_min", "e_max", "flux", "flux_err"]
[11]:
time_min | time_max | e_min [1] | e_max [1] | flux [1] | flux_err [1] |
---|---|---|---|---|---|
TeV | TeV | 1 / (cm2 s) | 1 / (cm2 s) | ||
float64 | float64 | float64 | float64 | float64 | float64 |
53343.922340092584 | 53343.94186555555 | 1.191457161449437 | 10.000000000000002 | 2.038021021453472e-11 | 2.120583429884751e-12 |
53343.954215092584 | 53343.97369425925 | 1.191457161449437 | 10.000000000000002 | 2.0610953064401673e-11 | 2.1406685569472896e-12 |
53345.96198129629 | 53345.98149518518 | 1.191457161449437 | 10.000000000000002 | 2.180692935101118e-11 | 2.7893729758578242e-12 |
53347.91319657407 | 53347.932710462956 | 1.191457161449437 | 10.000000000000002 | 2.5084814277817022e-11 | 2.914165879735225e-12 |
Running the light curve extraction in 1D¶
Building the 1D analysis configuration¶
[12]:
conf_1d = AnalysisConfig()
Definition of the data selection¶
Here we use the Crab runs from the HESS DL3 data release 1
[13]:
conf_1d.observations.obs_ids = [23523, 23526, 23559, 23592]
Definition of the dataset geometry¶
[14]:
# We want a 1D analysis
conf_1d.datasets.type = "1d"
# We want to extract the data by observation and therefore to not stack them
conf_1d.datasets.stack = False
# Here we define the ON region and make sure that PSF leakage is corrected
conf_1d.datasets.on_region = dict(
frame="icrs",
lon=83.63308 * u.deg,
lat=22.01450 * u.deg,
radius=0.1 * u.deg,
)
conf_1d.datasets.containment_correction = True
# Finally we define the energy binning for the spectra
conf_1d.datasets.geom.axes.energy = dict(
min=0.7 * u.TeV, max=10 * u.TeV, nbins=5
)
conf_1d.datasets.geom.axes.energy_true = dict(
min=0.3 * u.TeV, max=20 * u.TeV, nbins=20
)
Run the 1D data reduction¶
[15]:
analysis_1d = Analysis(conf_1d)
analysis_1d.get_observations()
analysis_1d.get_datasets()
Setting logging config: {'level': 'INFO', 'filename': None, 'filemode': None, 'format': None, 'datefmt': None}
Fetching observations.
No HDU found matching: OBS_ID = 23523, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23526, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23559, HDU_TYPE = rad_max, HDU_CLASS = None
No HDU found matching: OBS_ID = 23592, HDU_TYPE = rad_max, HDU_CLASS = None
Number of selected observations: 4
Reducing spectrum datasets.
Creating the background Maker.
No background maker set. Check configuration.
Define the model to be used¶
Here we don’t try to fit the model parameters to the whole dataset, but we use predefined values instead.
[16]:
target_position = SkyCoord(ra=83.63308, dec=22.01450, unit="deg")
spectral_model = PowerLawSpectralModel(
index=2.702,
amplitude=4.712e-11 * u.Unit("1 / (cm2 s TeV)"),
reference=1 * u.TeV,
)
sky_model = SkyModel(spectral_model=spectral_model, name="crab")
# Now we freeze these parameters that we don't want the light curve estimator to change
sky_model.parameters["index"].frozen = True
We assign the model to be fitted to each dataset. We can use the same gammapy.modeling.models.SkyModel
as before.
[17]:
models = Models([sky_model])
analysis_1d.set_models(models)
Reading model.
Models
Component 0: SkyModel
Name : crab
Datasets names : None
Spectral model type : PowerLawSpectralModel
Spatial model type :
Temporal model type :
Parameters:
index (frozen) : 2.702
amplitude : 4.71e-11 +/- 0.0e+00 1 / (cm2 s TeV)
reference (frozen) : 1.000 TeV
Extracting the light curve¶
[18]:
lc_maker_1d = LightCurveEstimator(
energy_edges=[1, 10] * u.TeV, source="crab", reoptimize=False
)
lc_1d = lc_maker_1d.run(analysis_1d.datasets)
[19]:
lc_1d.geom.axes.names
[19]:
['energy', 'time']
[20]:
lc_1d.to_table(sed_type="flux", format="lightcurve")
[20]:
time_min | time_max | e_ref [1] | e_min [1] | e_max [1] | flux [1] | flux_err [1] | ts [1] | sqrt_ts [1] | npred [1,4] | npred_excess [1,4] | stat [1] | is_ul [1] | counts [1,4] | success [1] |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
TeV | TeV | TeV | 1 / (cm2 s) | 1 / (cm2 s) | ||||||||||
float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | float64 | bool | float64 | bool |
53343.922340092584 | 53343.94186555555 | 3.451749065980083 | 1.191457161449437 | 10.000000000000002 | 2.2143915492725492e-11 | 2.460434433816868e-12 | 9669.936813659744 | 98.33583687374478 | 80.99951140247454 .. nan | 80.99950408935547 .. nan | -344.4671901995785 | False | 81.0 .. nan | True |
53343.954215092584 | 53343.97369425925 | 3.451749065980083 | 1.191457161449437 | 10.000000000000002 | 1.8788302692955377e-11 | 2.229760760425012e-12 | 8464.48492145235 | 92.0026354049293 | nan .. nan | nan .. nan | -290.3078440983763 | False | nan .. nan | True |
53345.96198129629 | 53345.98149518518 | 3.451749065980083 | 1.191457161449437 | 10.000000000000002 | 2.155318731462195e-11 | 3.018049212497754e-12 | 6065.962231301234 | 77.88428744811905 | nan .. nan | nan .. nan | -194.3702461596488 | False | nan .. nan | True |
53347.91319657407 | 53347.932710462956 | 3.451749065980083 | 1.191457161449437 | 10.000000000000002 | 2.4853613061210656e-11 | 3.235664079237356e-12 | 7019.342401850264 | 83.78151587223917 | nan .. 58.999557457021695 | nan .. 58.99955749511719 | -226.71637982372326 | False | nan .. 59.0 | True |
Compare results¶
Finally we compare the result for the 1D and 3D lightcurve in a single figure:
[21]:
ax = lc_1d.plot(marker="o", label="1D")
lc_3d.plot(ax=ax, marker="o", label="3D")
plt.legend()
[21]:
<matplotlib.legend.Legend at 0x144c3de80>
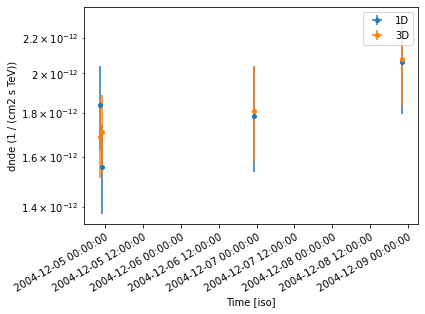
Night-wise LC estimation¶
Here we want to extract a night curve per night. We define the time intervals that cover the three nights.
[22]:
time_intervals = [
Time([53343.5, 53344.5], format="mjd", scale="utc"),
Time([53345.5, 53346.5], format="mjd", scale="utc"),
Time([53347.5, 53348.5], format="mjd", scale="utc"),
]
To compute the LC on the time intervals defined above, we pass the LightCurveEstimator
the list of time intervals.
Internally, datasets are grouped per time interval and a flux extraction is performed for each group.
[23]:
lc_maker_1d = LightCurveEstimator(
energy_edges=[1, 10] * u.TeV,
time_intervals=time_intervals,
source="crab",
reoptimize=False,
selection_optional="all",
)
nightwise_lc = lc_maker_1d.run(analysis_1d.datasets)
nightwise_lc.plot(color="tab:orange")
ax = nightwise_lc.plot_ts_profiles()
ax.set_ylim(1e-12, 3e-12);
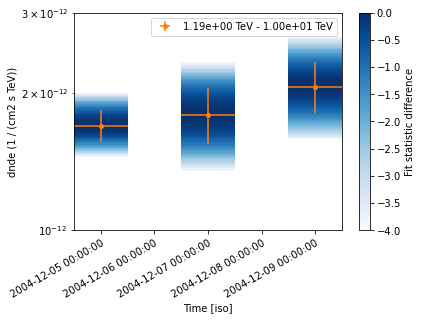
What next?¶
When sources are bright enough to look for variability at small time scales, the per-observation time binning is no longer relevant. One can easily extend the light curve estimation approach presented above to any time binning. This is demonstrated in the following tutorial which shows the extraction of the lightcurve of an AGN flare.
[ ]: